Debug ECL programming
In the ECL language, operations such as OUTPUT cannot be performed in the definition, complicating our view of intermediate variables. This blog introduces debugging tips for several ECL programming language.
Use WHEN to output intermediate variables
Unlike programming languages such as C++ and Python, in ECL, the
OUTPUT
operation cannot be performed in the function
definition. It will prompt
WHEN must be used to associate an action with a definition
We can use WHEN
to output intermediate variables. For
example:
1 | //a FUNCTION with side-effect Action |
For multiple outputs, SEQUENTIAL
can be used. For
example:
1 | //a FUNCTION with side-effect Action |
Logging with Std.System.Log
By using Std.System.Log
, some logs can be recorded. For
example:
1 | IMPORT Std.System.Log AS Syslog; |
In Helpers of ECL Watch, in EclAgentLog
, you can find
the corresponding log.
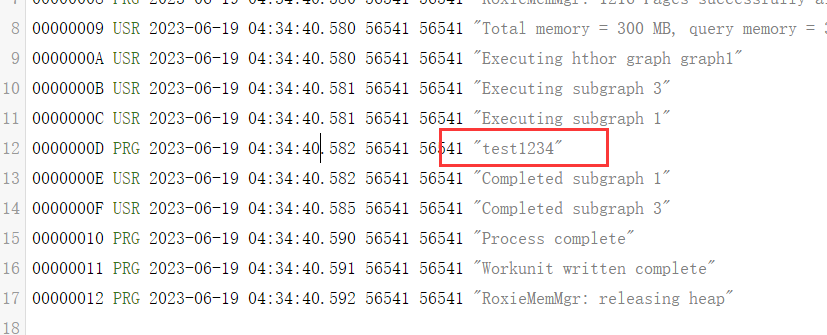
In embedded languages, use assert to check intermediate variables
When we need to debug some embedded Python codes, we cannot use the
print
function to print intermediate variables and can only
output them externally by means of an
assert False, 'message'
. For example, if I want to view the
summary of the model I defined, I can use the following:
1 | # Debug |